Flow Control
Flow Control:
Flow Control describes the order in which the statements will be executed at runtime.
a) Selection Statements :Conditional statements also Known as Selection Statement, are used to make decision on given condition. If condition is true, set of statement is executed, otherwise other statement is executed.
1) if Statement:Selects and executes the statement based on given condition.
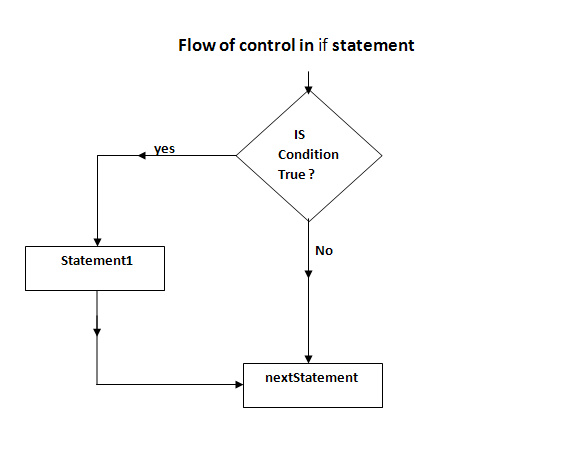
Syntax:
For a single Statement:- if(condition)
{
statement1;
nextstatement;
For a set of statement:-
if(condition)
{
statement1;
statement2;
}
nextstatement;
For a single Statement:- if(condition)
{
statement1;
nextstatement;
For a set of statement:-
if(condition)
{
statement1;
statement2;
}
nextstatement;
Example: Create class if_statement to demonstrate the use of if statement
2) If - else : One of two possible statements to execute depending upon the result of condition.
Syntax :
if(condition)
{
statement1;
}
else
{
statement2;
}
if(condition)
{
statement1;
}
else
{
statement2;
}
Example: Create class if_statement to demonstrate the use of if-else statement
3) Switch Statement: It selects a set of statement from available sets of statement. The switch statement evaluates the value of an expression and compare it with the list of integer,char, short ,byte constants.when match found all the statement associated with that constant are executed.
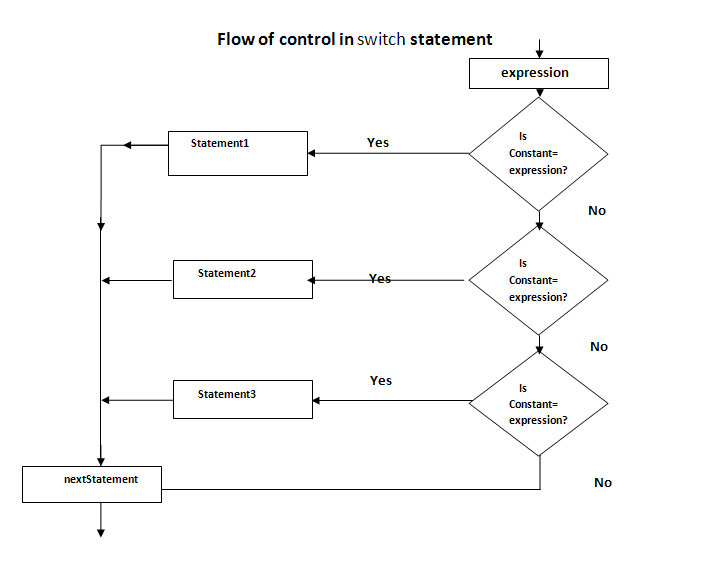
Syntax:
Switch(x)
{
Case1:statement1;
break;
Case2:statement2;
break;
case3:statement3;
break;
default:statement4;
}
nextstatement;
Switch(x)
{
Case1:statement1;
break;
Case2:statement2;
break;
case3:statement3;
break;
default:statement4;
}
nextstatement;
Example: Create class switch_statement to demonstrate the use of Switch statement
b) Iterative Statements:The statement that cause a set of statement to be executed repeatedly either for a specific number of times or until number of condition is satisfied are known as iteration statement. The various iteration statement used in java are for loop, while loop, do-while loop.
1) While:-
If we don't know the no. of iteration in advance then the best suitable loop is while loop.
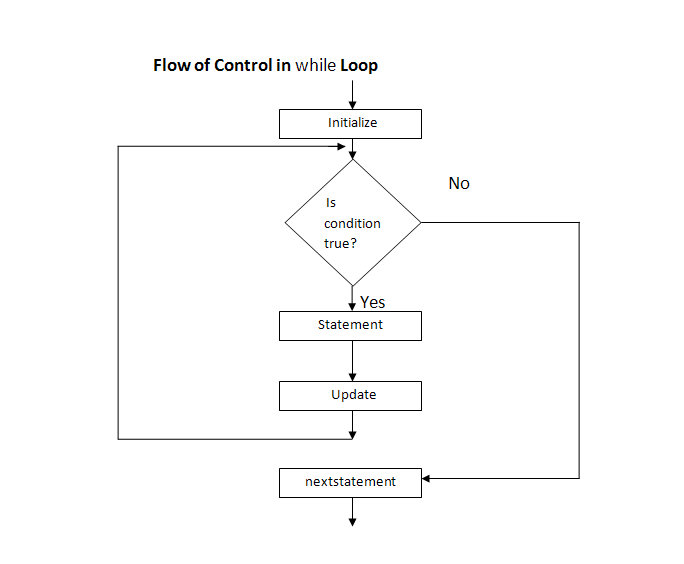
Syntax:-
while(condition)
{
// body of loop
}
while(condition)
{
// body of loop
}
Example: Create class while_loop to demonstrate the use of while statement
2) do-while: If the body of loop is to be executed at least once ,no matter whether the initial state of the condition is true or false ,do-while loop is used.

Syntax:-
do
{
//body of do-while loop
}
while(condition);
do
{
//body of do-while loop
}
while(condition);
Example: Create class do_while to demonstrate the use of do-while statement
3) for loop: The for loop is one of the most widely used loop in java. The for loop is deterministic loop, that is number of time the body of loop is executed is known in advance.

Syntax:-
for(initialize; condition ; increment/decrement)
{
//body of loop
}
for(initialize; condition ; increment/decrement)
{
//body of loop
}
Example: Create class for_loops to demonstrate the use of for loop